Introduction
This is an example guide to setting up Modbus TCP communication between a client and server using CONTROLLINO and an Ethernet shield. This tutorial will help you configure the client to read and write inputs and outputs and the server to synchronize its state with the client.
Prerequisites
- Installed Arduino IDE.
- CONTROLLINO PLC board (e.g., MAXI(also Automation), or MEGA).
- Basic understanding of Modbus TCP and Ethernet setup.
Modbus TCP connection example
Point to point connection
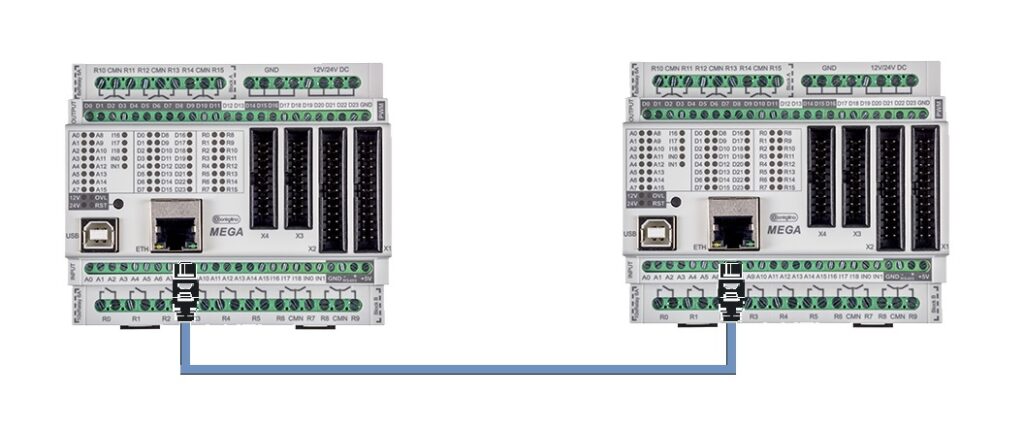
Connection using switch
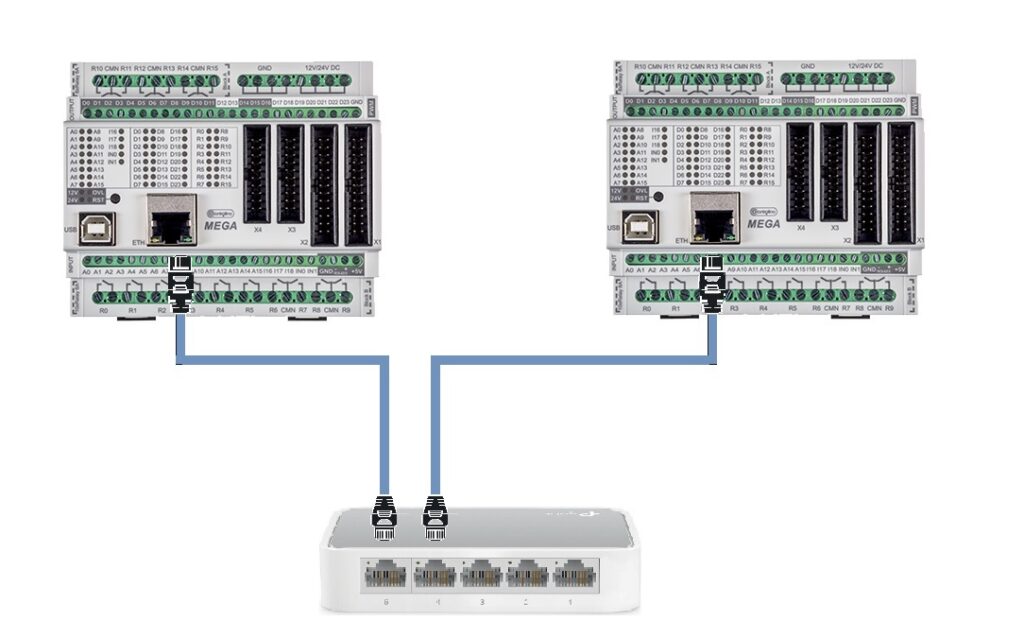
Example Code
You can download the example Modbus TCP Client and Server code from the following GitHub repository:
- Modbus TCP Client code
- Modbus TCP Server code
- Detailed example explanation
Step-by-Step Guide
Modbus TCP Client
The Modbus TCP client communicates with the server to toggle coils and read and write registers.
Step 1: Setup the Arduino Environment
- Connect your CONTROLLINO board to your computer.
- Open the Arduino IDE.
- Ensure that the CONTROLLINO is selected in the
Tools
menu. - Include the necessary libraries for communication:
ArduinoRS485
ArduinoModbus
Controllino
SPI
Ethernet
Step 2: Initialize the Ethernet and Modbus TCP Client
In the setup()
function:
- Start the Ethernet connection by calling
Ethernet.begin(mac, ip)
using the MAC address of your Ethernet shield. - Check the Ethernet hardware and cable connection.
- If the connection is successful, initialize the Modbus TCP client by using
modbusTCPClient.begin(server, 502)
.
Step 3: Configure Digital and Analog Pins
In the loop()
function:
- Digital Inputs and Coils:
- Declare an array
slaveInputs[]
to store the state of the slave’s digital inputs. - Read these inputs using
modbusTCPClient.discreteInputRead()
. - Synchronize the state of the slave inputs to the master outputs using
digitalWrite()
. - Write the current state of the master digital input to the slave using
modbusTCPClient.coilWrite()
.
- Declare an array
- Analog Inputs and Holding Registers:
- Declare an array
slaveInRegisters[]
to store the state of the slave’s holding registers. - Read the analog inputs using
modbusTCPClient.inputRegisterRead()
. - Synchronize the master’s analog outputs using
analogWrite()
and update the slave’s holding registers usingmodbusTCPClient.holdingRegisterWrite()
.
- Declare an array
Modbus TCP Server
The Modbus TCP server simulates a coil and synchronizes the state of its inputs and outputs with the client.
Step 1: Setup the Arduino Environment
- Connect your CONTROLLINO board to your computer.
- Open the Arduino IDE.
- Ensure that the CONTROLLINO is selected in the
Tools
menu. - Include the same libraries as in the client setup:
ArduinoRS485
ArduinoModbus
Controllino
SPI
Ethernet
Step 2: Initialize the Ethernet and Modbus TCP Server
In the setup()
function:
- Start the Ethernet connection using
Ethernet.begin(mac, ip)
. - Start the Modbus TCP server by calling
modbusTCPServer.begin()
. - Configure the Modbus coils, discrete inputs, holding registers, and input registers using:
modbusTCPServer.configureCoils()
modbusTCPServer.configureDiscreteInputs()
modbusTCPServer.configureHoldingRegisters()
modbusTCPServer.configureInputRegisters()
Step 3: Configure Digital and Analog Pins
- Set up digital input and output pins.
- Use
pinMode()
to define the pins as either INPUT or OUTPUT. - Initialize output pins to a LOW state.
- Use
- Set up analog output pins for holding registers using
analogWrite()
.
Step 4: Synchronizing Coils and Registers
In the loop()
function:
- Client Connection:
- Wait for incoming client connections using
ethernetServer.available()
. - Accept the client connection with
modbusTCPServer.accept(client)
.
- Wait for incoming client connections using
- Synchronize Coils and Registers:
- In the
executeSynchronization()
function:- Read the state of the server’s coils using
modbusTCPServer.coilRead()
, and update the corresponding output pins usingdigitalWrite()
. - Read the state of the input pins using
digitalRead()
and update the corresponding coil register withmodbusTCPServer.coilWrite()
. - For analog outputs, write the value from the holding register to the PWM outputs using
analogWrite()
. - Read the analog input pins and update the corresponding input registers using
modbusTCPServer.inputRegisterWrite()
.
- Read the state of the server’s coils using
- In the
Conclusion
You have now successfully configured Modbus TCP communication between a CONTROLLINO client and server using an Ethernet shield. The client reads and writes digital and analog inputs/outputs, while the server synchronizes its state accordingly. Experiment with different pin configurations and data handling to expand your project!